AndyB
Well-known member
I have an add-on that I'm creating. The add-on shows a calendar list like this:
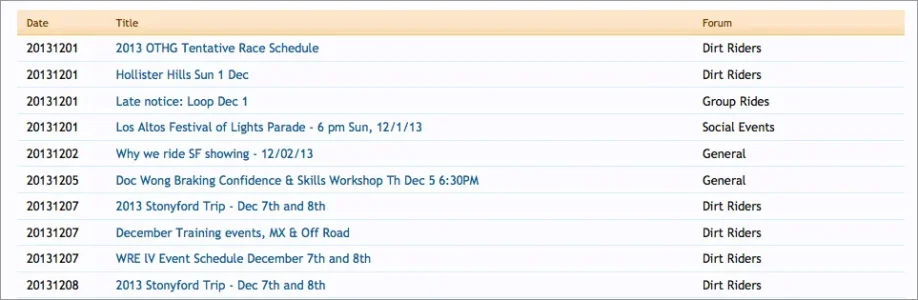
As you can see the date format is a bit difficult to read.
What functions are available in the template system to make the date format easier to read in this situation?
Here's the template code:
I would like to be able to show the date with separators like 2013/12/08 or 12/08/2013.
Thank you.
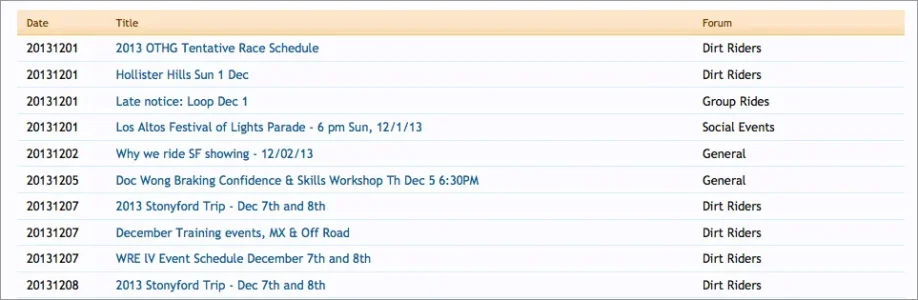
As you can see the date format is a bit difficult to read.
What functions are available in the template system to make the date format easier to read in this situation?
Here's the template code:
Code:
{xen:phrase calendar}
<br /><br />
<table class="dataTable">
<tr class="dataRow">
<th>{xen:phrase date}</th>
<th>{xen:phrase title}</th>
<th>{xen:phrase forum}</th>
</tr>
<xen:foreach loop="$threads" value="$thread">
<tr class="dataRow">
<td>{$thread.calendar_date}</td>
<td><a href="{xen:link 'threads', $thread}" />{$thread.title}</a></td>
<td>{$thread.nodeTitle}</td>
</tr>
</xen:foreach>
</table>
I would like to be able to show the date with separators like 2013/12/08 or 12/08/2013.
Thank you.
Last edited: