Gossamer
Active member
I'm currently working on an input form that creates an array of urls. However, it's apparently saving a bunch of empty fields into that array that I don't want.
This is my template code:
My ControllerAdmin:
And this is what it looks like:
How can I make sure it's not saving information from the empty fields?
This is my template code:
Code:
<xen:title>{xen:phrase goss_member_awards_of_x, 'username={$user.username}'}</xen:title>
<xen:form action="{xen:adminlink 'member-awards/save', $user}" class="AutoValidator" data-redirect="on">
<fieldset>
<xen:controlunit label="{xen:phrase goss_member_award_link}:">
<ul class="AwardList">
<xen:foreach loop="$memberAwardUrls" key="$choice" value="$text">
<li>
<input type="text" name="goss_memberaward_urls[]" value="{$text}" class="textCtrl" placeholder="{xen:phrase image_url}" size="50" />
</li>
</xen:foreach>
<li>
<input type="text" name="goss_memberaward_urls[]" value="{$text}" class="textCtrl" placeholder="{xen:phrase image_url}" size="50" />
</li>
</ul>
<input type="button" value="{xen:phrase goss_member_awards_add_additional_url}" class="button smallButton FieldAdder" data-source="ul.AwardList li" />
</xen:controlunit>
</fieldset>
<xen:submitunit save="{xen:phrase save_field}">
</xen:submitunit>
</xen:form>
My ControllerAdmin:
PHP:
public function actionUser()
{
$userId = $this->_input->filterSingle('user_id', XenForo_Input::UINT);
$userModel = $this->_getUserModel();
$user = $userModel->getUserById($userId);
$urls = $user['goss_memberaward_urls'];
if (!is_array($urls))
{
$urls = ($urls ? XenForo_Helper_Php::safeUnserialize($urls) : array());
}
$viewParams = array(
'user' => $user,
'memberAwardUrls' => $urls
);
return $this->responseView(null, 'goss_memberawards_user', $viewParams);
}
public function actionSave()
{
$userId = $this->_input->filterSingle('user_id', XenForo_Input::UINT);
$awardurl = $this->_input->filter(array(
'goss_memberaward_urls' => XenForo_Input::ARRAY_SIMPLE
));
$dw = XenForo_DataWriter::create('XenForo_DataWriter_User');
$dw->setExistingData($userId);
$dw->bulkSet($awardurl);
$dw->save();
//Send a response to the user, so he know that everything went fine with this action
return $this->responseRedirect(
XenForo_ControllerResponse_Redirect::SUCCESS,
$this->getDynamicRedirect()
);
}
And this is what it looks like:
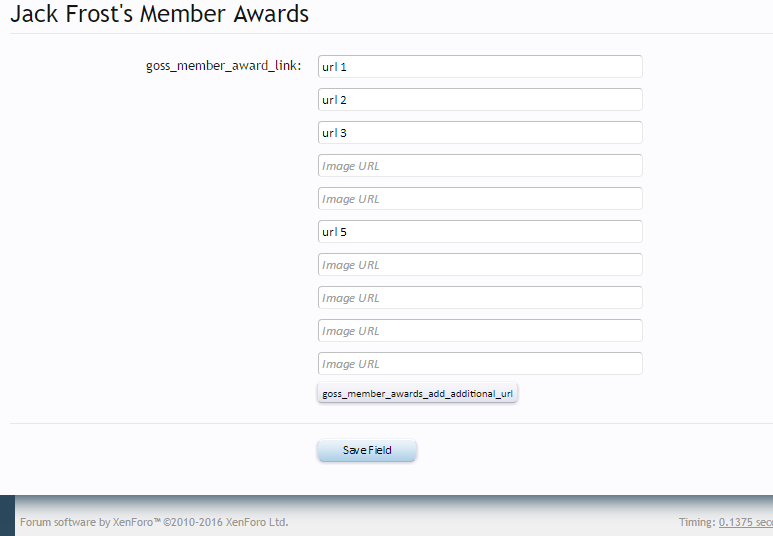
How can I make sure it's not saving information from the empty fields?