AndyB
Well-known member
In regards to the new API and the introduction here:
https://xenforo.com/community/threads/rest-api.155632/
What are the first steps to using the API? In the Admin control panel I created an API key.
I assume I should be able to put in the address bar of my browser the following example to retrieve information about thread_id 123:
But this results in the standard error message:
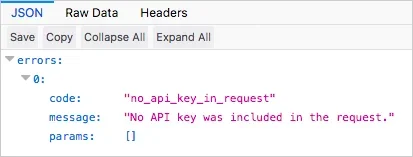
Thank you.
https://xenforo.com/community/threads/rest-api.155632/
What are the first steps to using the API? In the Admin control panel I created an API key.
I assume I should be able to put in the address bar of my browser the following example to retrieve information about thread_id 123:
https://www.domain.com/api/threads/123/?key={my api key}
But this results in the standard error message:
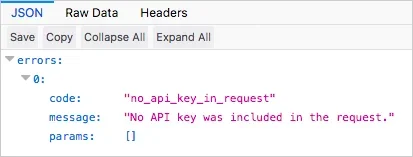
Thank you.