AndyB
Well-known member
Excellent suggestions, Marcus.
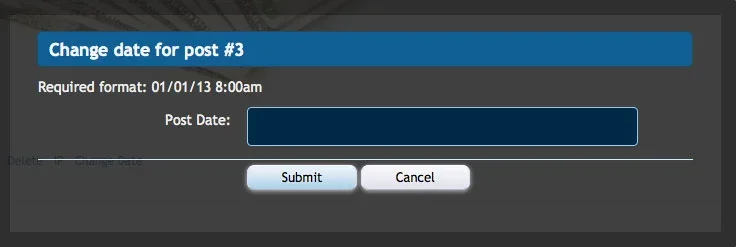
Post.php
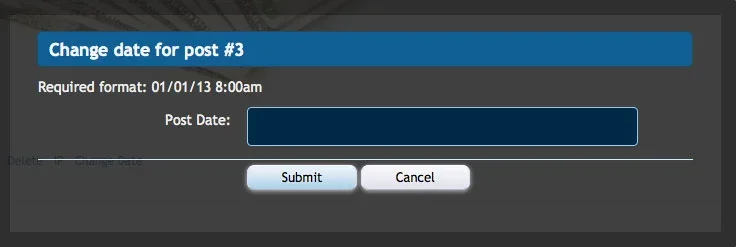
Post.php
PHP:
<?php
class Andy_ChangeDate_ControllerPublic_Post extends XFCP_Andy_ChangeDate_ControllerPublic_Post
{
public function actionChangedate()
{
// if you are not a super admin, return
if (!XenForo_Visitor::getInstance()->isSuperAdmin())
{
return;
}
// get post_id from route
$postId = $this->_input->filterSingle('post_id', XenForo_Input::UINT);
// get all post data
$post = $this->getModelFromCache('XenForo_Model_Post')->getPostById($postId);
$viewParams = array('post' => $post);
// display andy_changedate template overlay
return $this->responseView('Andy_DateChange_ViewPublic_Post','andy_changedate',$viewParams);
}
public function actionChangedatesave()
{
// make sure data comes from $_POST
$this->_assertPostOnly();
// if you are not a super admin, you will get an error
if (!XenForo_Visitor::getInstance()->isSuperAdmin())
{
return;
}
$post['post_id'] = $this->_input->filterSingle('post_id', XenForo_Input::UINT);
// get new post date from input text
$newPostDate = $this->_input->filterSingle('new_post_date', XenForo_Input::STRING);
// convert to unix timestamp
$dateline = strtotime($newPostDate ) - XenForo_Locale::getTimeZoneOffset();
if (!$dateline)
{
return $this->responseError(new XenForo_Phrase('incorrect_date_format_entered'));
}
//########################################
// start database operations
//########################################
// update the xf_post.post_date
$dw = XenForo_DataWriter::create('XenForo_DataWriter_DiscussionMessage_Post');
$dw->setExistingData($post['post_id']);
$dw->set('post_date', $dateline);
$dw->save();
// update the xf_post.position for each post in the thread
// also updates other tables with first post and last post information
$threadId = $dw->get('thread_id');
$dw = XenForo_DataWriter::create('XenForo_DataWriter_Discussion_Thread');
$dw->setExistingData($threadId);
$dw->rebuildDiscussion();
$dw->save();
//########################################
// return with response redirect
//########################################
return $this->responseRedirect(
XenForo_ControllerResponse_Redirect::SUCCESS,
XenForo_Link::buildPublicLink('posts', $post),'Post Date Changed');
}
}
?>