AndyB
Well-known member
In one of my add-ons I have a need in the Options to be able to select forums. The following code works very well with the exception that nodes besides Forums can be selected. I would like to make it so that nodes which are a Category, Page or LinkForum cannot be selected, only Forums should be able to be selected.
In this example "Community" is a Category:
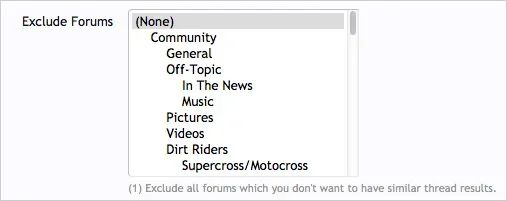
Options page code:
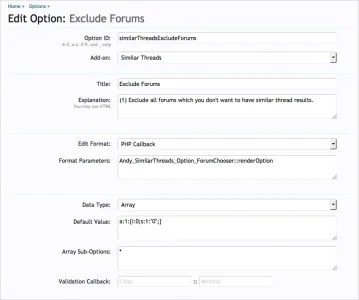
Folder and Files
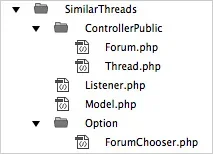
ForumChooser.php
Admin Template -> andy_option_list_option_select
In this example "Community" is a Category:
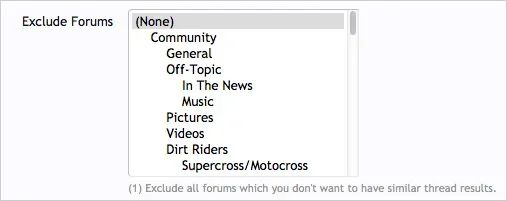
Options page code:
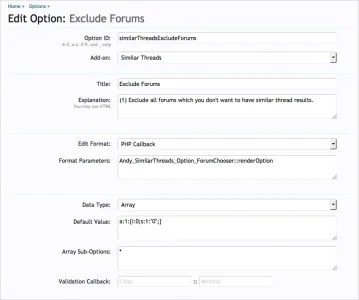
Folder and Files
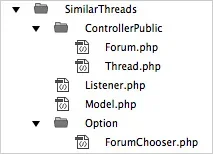
ForumChooser.php
PHP:
<?php
abstract class Andy_SimilarThreads_Option_ForumChooser
{
public static function renderOption(XenForo_View $view, $fieldPrefix, array $preparedOption, $canEdit)
{
$value = $preparedOption['option_value'];
$editLink = $view->createTemplateObject('option_list_option_editlink', array(
'preparedOption' => $preparedOption,
'canEditOptionDefinition' => $canEdit
));
$nodeModel = XenForo_Model::create('XenForo_Model_Node');
$forumOptions = $nodeModel->getNodeOptionsArray($nodeModel->getAllNodes(), $preparedOption['option_value'], '(None)');
return $view->createTemplateObject('andy_option_list_option_select', array(
'fieldPrefix' => $fieldPrefix,
'listedFieldName' => $fieldPrefix . '_listed[]',
'preparedOption' => $preparedOption,
'formatParams' => $forumOptions,
'editLink' => $editLink
));
}
}
Admin Template -> andy_option_list_option_select
Code:
<xen:selectunit label="{$preparedOption.title}" name="{$fieldPrefix}[{$preparedOption.option_id}]" value="{xen:raw $preparedOption.option_value}"
hint="{$preparedOption.hint}" size="10" multiple="true">
<xen:options source="$formatParams" />
<xen:explain>{xen:raw $preparedOption.explain}</xen:explain>
<xen:html>
<input type="hidden" name="{$listedFieldName}" value="{$preparedOption.option_id}" />
{xen:raw $editLink}
</xen:html>
</xen:selectunit>