developr
Active member
I want to add avatars to users via the xenforo api.
The API will response with "success" but it doesn't work.
The API require "file" in postdata. But how? A link to the file doesn't work. A resource doesn't work. Without an error it's hard to find a solution.
xenforo.com
PHP:
function apiCall($action,$scope,$post,$upload=0) {
$headers = array(
'Content-type: application/x-www-form-urlencoded',
'XF-Api-User: 1',
'XF-Api-Key: '.APIKEY
);
if(!$upload) {
$post = @http_build_query($post);
}
$url = 'https://127.0.0.1/api'.$scope;
$ch = curl_init();
if($upload) {
$file = $post['avatar'];
$mime = mime_content_type($file);
$info = pathinfo($file);
$name = $info['basename'];
$output = new CURLFile($file, $mime, $name);
$post['avatar'] = $output;
}
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POSTFIELDS,$post);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST,$action);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$json = curl_exec($ch);
curl_close($ch);
return json_decode($json);
}
The API will response with "success" but it doesn't work.
The API require "file" in postdata. But how? A link to the file doesn't work. A resource doesn't work. Without an error it's hard to find a solution.
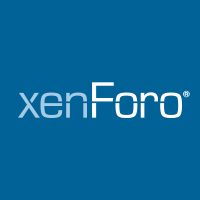
REST API endpoints (2.2)
This document assumes you have already created an API key and setup the necessary headers for your request. Learn more about using the API.
