asprin
Active member
As part of my addon, I have a brand new table called
Now in order for the
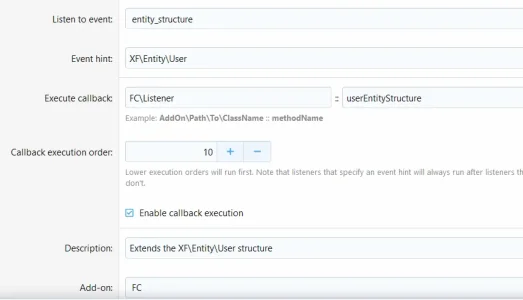
Code inside Listener.php file is as follows:
Now, when I use the following to fetch all events:
I'm getting the following error:
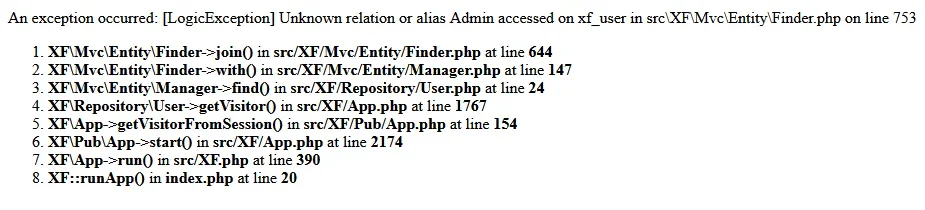
What am I doing wrong here?
If it helps,
xf_fc_events
. In this table, there is a column named created_by
in which I will be storing the logged in user's user_id.Now in order for the
XF:User
entity to be aware of this relation, I created a code_event_listener
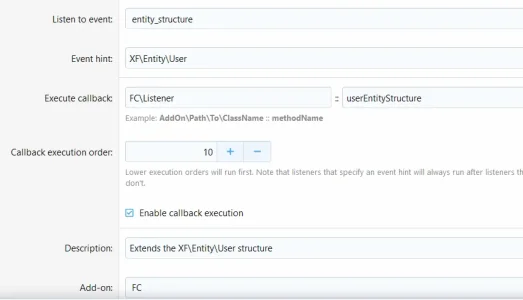
Code inside Listener.php file is as follows:
PHP:
namespace FC;
use XF\Mvc\Entity\Entity;
class Listener
{
public static function userEntityStructure(\XF\Mvc\Entity\Manager $em, \XF\Mvc\Entity\Structure &$structure)
{
$structure->relations = [
'EventCreator' => [
'entity' => 'FC:Events',
'type' => Entity::TO_MANY, // one user can create multiple events
'conditions' => 'user_id',
'key' => 'created_by'
]
];
}
}
Now, when I use the following to fetch all events:
PHP:
<?php
namespace FC\Repository;
use XF\Mvc\Entity\Finder;
use XF\Mvc\Entity\Repository;
class Events extends Repository
{
/**
* @return Finder
*/
public function fetchAllEvents()
{
$visitor = \XF::visitor();
$finder = $this->finder('FC:Events');
$finder
->setDefaultOrder('sys_created_on', 'DESC')
->with('User', true);
return $finder;
}
}
I'm getting the following error:
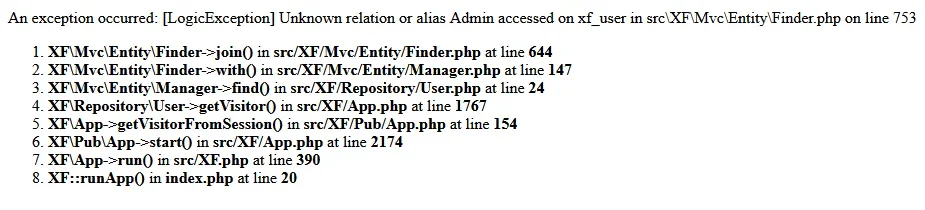
What am I doing wrong here?
If it helps,
xf_fc_events
currently has only 1 record and created_by
column value is "1" which is an admin account with username "admin"