Snog
Well-known member
In the type of add-on you're doing, in the beginning you will have a larger number of writes to your table. It's unavoidable because each time the highest number of users changes to a higher value, you'll have to write that value to your table. Once the number stabilizes, it would usually only be an occasional write, if it ever gets written again at all.
And so far as your theory about all add-ons creating queries (if I understood you correctly) you're wrong. Here's a comparison with one of my add-ons turned off and turned on, look at the query counts...
Add-on off...
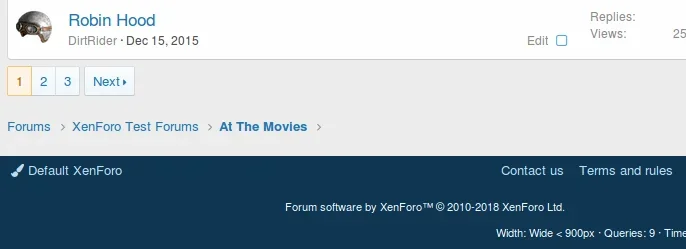
Add-on on...
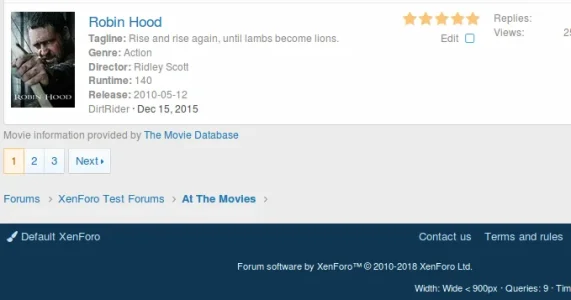
I did not add any fields to the thread table. That is entirely with a separate table containing the movie info. No queries added.
And so far as your theory about all add-ons creating queries (if I understood you correctly) you're wrong. Here's a comparison with one of my add-ons turned off and turned on, look at the query counts...
Add-on off...
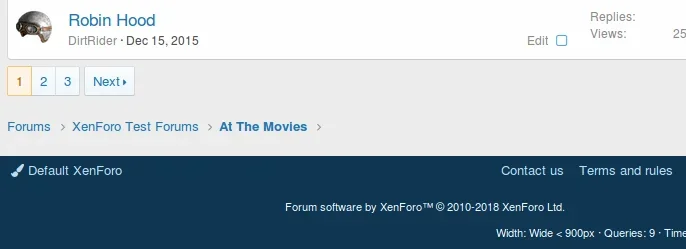
Add-on on...
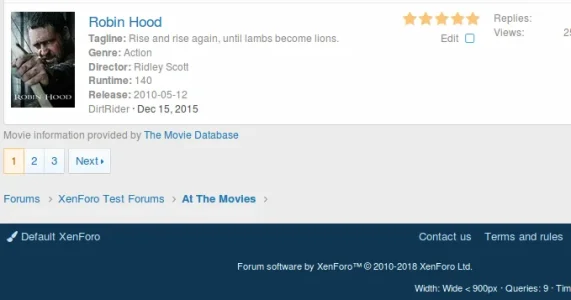
I did not add any fields to the thread table. That is entirely with a separate table containing the movie info. No queries added.
Last edited: