AndyB
Well-known member
In an add-on I'm creating, I would like to allow an easy way to override the time limit for editing a post. So it looks like I need to extend the canEdit function located here:
src/XF/Entity/Post.php
The code I'm wanting to extend is this:
My add-on has a file called Listener.php
This is the Code event listener
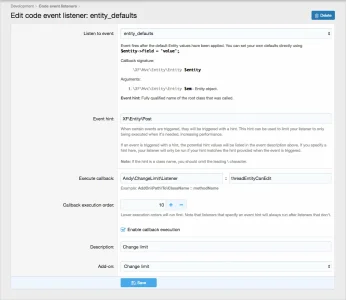
When I click the Edit link below a post I'm expecting to see the error message due to the echo command, but this is not working due to me not understanding how to properly extend the canEdit function.
src/XF/Entity/Post.php
The code I'm wanting to extend is this:
PHP:
public function canEdit(&$error = null)
{
$thread = $this->Thread;
$visitor = \XF::visitor();
if (!$visitor->user_id || !$thread)
{
return false;
}
if (!$thread->discussion_open && !$thread->canLockUnlock())
{
$error = \XF::phraseDeferred('you_may_not_perform_this_action_because_discussion_is_closed');
return false;
}
$nodeId = $thread->node_id;
if ($visitor->hasNodePermission($nodeId, 'editAnyPost'))
{
return true;
}
if ($this->user_id == $visitor->user_id && $visitor->hasNodePermission($nodeId, 'editOwnPost'))
{
$editLimit = $visitor->hasNodePermission($nodeId, 'editOwnPostTimeLimit');
if ($editLimit != -1 && (!$editLimit || $this->post_date < \XF::$time - 60 * $editLimit))
{
$error = \XF::phraseDeferred('message_edit_time_limit_expired', ['minutes' => $editLimit]);
return false;
}
if (!$thread->Forum || !$thread->Forum->allow_posting)
{
$error = \XF::phraseDeferred('you_may_not_perform_this_action_because_forum_does_not_allow_posting');
return false;
}
return true;
}
return false;
}
My add-on has a file called Listener.php
PHP:
<?php
namespace Andy\ChangeLimit;
use XF\Mvc\Entity\Entity;
class Listener
{
public static function threadEntityCanEdit(\XF\Mvc\Entity\Entity $em)
{
echo 'test';
}
}
This is the Code event listener
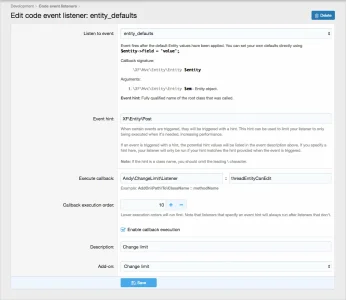
When I click the Edit link below a post I'm expecting to see the error message due to the echo command, but this is not working due to me not understanding how to properly extend the canEdit function.
Last edited: