AndyB
Well-known member
I have an Options field called Category. For example the Option field has the following:
Accessories,Cameras,Electronics
In my PHP code I convert this text field to a simple array.
What I would like to be able to do is is create a drop down in a template that allows me to select any of these three items:
(example of dropdown)
My PHP code looks like this:
The above PHP code is incorrect. $results needs to be a multidimensional array. Normally this is easy to create because I would normally query a database table and use the fetchAll command which creates a multidimensional array. But I don't want to create a database table to store my categories, rather I want to use a simple Option and store the categories as a comma separated text.
How can I easily convert a simple array to a multidimensional array that is in the correct format to use in a xen:foreach loop in a template?
Thank you.
Accessories,Cameras,Electronics
In my PHP code I convert this text field to a simple array.
What I would like to be able to do is is create a drop down in a template that allows me to select any of these three items:
(example of dropdown)
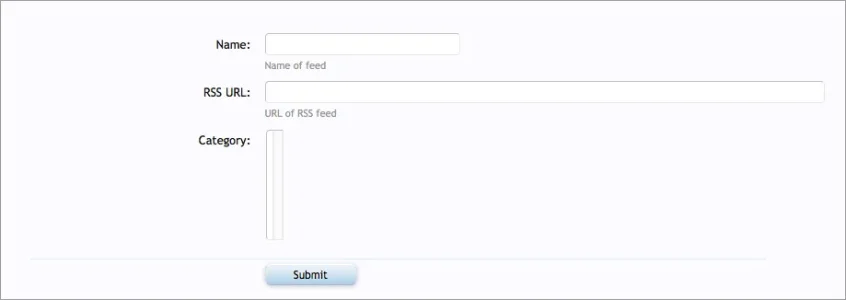
My PHP code looks like this:
PHP:
// get options from Admin CP -> Options -> Ebay -> Category
$category = XenForo_Application::get('options')->ebayCategory;
// put into array
$categoryArray = explode(',', $category);
$results = $categoryArray;
// prepare viewParams
$viewParams = array(
'results' => $results
);
// send to template
return $this->responseView('Andy_Ebay_ViewPublic_Ebay', 'andy_ebay_add');
The above PHP code is incorrect. $results needs to be a multidimensional array. Normally this is easy to create because I would normally query a database table and use the fetchAll command which creates a multidimensional array. But I don't want to create a database table to store my categories, rather I want to use a simple Option and store the categories as a comma separated text.
How can I easily convert a simple array to a multidimensional array that is in the correct format to use in a xen:foreach loop in a template?
Thank you.