DeltaHF
Well-known member
I'm trying to create an add-on which modified what happens when a user requests an account confirmation email. After some searching, I think I need to override actionResend() in /library/XenForo/ControllerPublic/AccountConfirmation.php.
I've never made a XenForo add-on before, and I'm trying to keep it as simple as possible while getting my feet wet. I've created the following files in a /library/DeltaHF directory:
/library/DeltaHF/ActivationMod.php
/library/DeltaHF/Listener.php
I then defined a new Event Listener as follows:
So far, so good, until I actually try to re-send an activation email. That throws an error warning on the page and puts the following Fatal Error into the Server Error Log:
I've never made a XenForo add-on before, and I'm trying to keep it as simple as possible while getting my feet wet. I've created the following files in a /library/DeltaHF directory:
/library/DeltaHF/ActivationMod.php
PHP:
<?php
class DeltaHF_ActivationMod extends XFPC_DeltaHF_ActivationMod
{
public function actionResend()
{
// send an email to let me know we made it in here
mail("my_email_address@example.com", "Extension Test", "It worked!");
return parent::actionResend();
}
}
/library/DeltaHF/Listener.php
PHP:
<?php
class DeltaHF_Listener
{
public static function loadClassListener($class, array &$extend)
{
if ($class == 'XenForo_ControllerPublic_AccountConfirmation')
{
$extend[] = 'DeltaHF_ActivationMod';
}
}
}
I then defined a new Event Listener as follows:
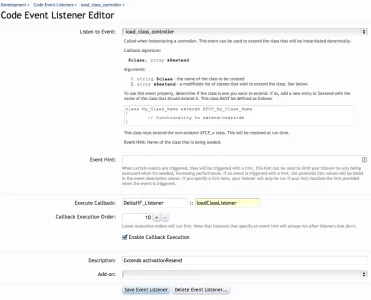
So far, so good, until I actually try to re-send an activation email. That throws an error warning on the page and puts the following Fatal Error into the Server Error Log:
What am I doing wrong? I can tell it has to be something simple, but I'm lost.ErrorException: Fatal Error: Class 'XFPC_DeltaHF_ActivationMod' not found - library/DeltaHF/ActivationMod.php:10