D
Deleted member 110473
Guest
I shared this code in another thread, I have a working implementation but have a question regarding how to improve it:
Location: src/addons/Pages/Providers.php
And in the nodes, I created a page and using the php callback provided within the page node I have:
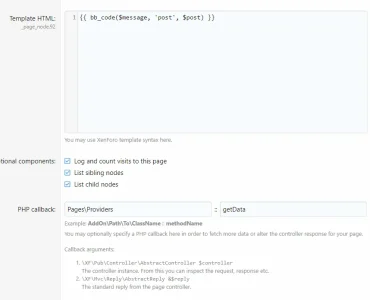
Now, what I am stuck at (as you can probably tell from the code) is that
So, lets say I create a new page, I want that new page to have
Location: src/addons/Pages/Providers.php
PHP:
<?php
/*
* This file is part of a XenForo add-on.
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Pages;
/**
* Static methods for the Providers page.
*/
class Providers
{
/**
* @param \XF\Pub\Controller\AbstractController $controller
* @param \XF\Mvc\Reply\AbstractReply &$reply
*/
public static function getData(
\XF\Pub\Controller\AbstractController $controller,
\XF\Mvc\Reply\AbstractReply &$reply
) {
$active = 1;
if ($reply instanceof \XF\Mvc\Reply\View) {
$finder = \XF::finder('XF:Thread');
$thread = $finder->where('thread_id', $active)->fetchOne();
$firstPost = \XF::app()->finder('XF:Post')->where('post_id', $thread['first_post_id'])->fetchOne();
$viewParams = [
'title' => $thread['title'],
'message' => $firstPost['message']
];
$reply->setParams($viewParams);
}
}
}
And in the nodes, I created a page and using the php callback provided within the page node I have:
Pages\Providers
as my class and getData
as my method. The following screenshot shows the setup: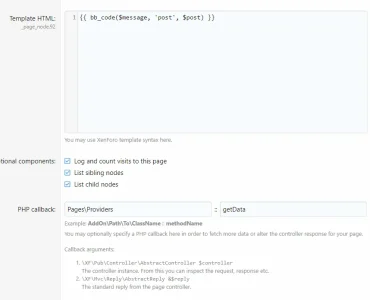
Now, what I am stuck at (as you can probably tell from the code) is that
$active
is a static variable set to be thread_id=1, so I can only display first post of thread 1 in the page. What I would like is to reuse the code again but only "send" the thread_id within the xenforo page to automatically get the post I really wanna display.So, lets say I create a new page, I want that new page to have
$active=2
and show the first post of thread_id=2. Is this possible using the PHP callback provided within pages?