AndyB
Well-known member
Purpose:
The purpose of this thread is to provide additional information on the History v1.0 add-on.
To download and install this add-on please visit the following XenForo Resource:
http://xenforo.com/community/resources/history.2653/
Description:
This add-on will allow members to quickly return to previously viewed threads.
Features:
(Example of History page)
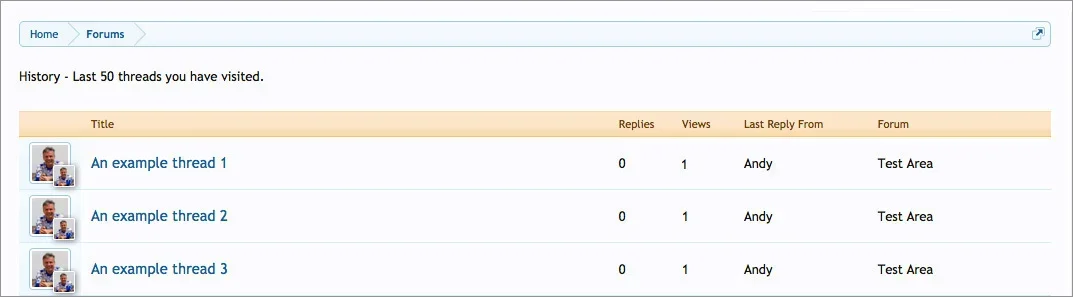
The purpose of this thread is to provide additional information on the History v1.0 add-on.
To download and install this add-on please visit the following XenForo Resource:
http://xenforo.com/community/resources/history.2653/
Description:
This add-on will allow members to quickly return to previously viewed threads.
Features:
- Shows a list of last viewed threads
- Highlights threads with new posts
- Places History link in navigation bar for easy access
- Fully phrased

(Example of History page)
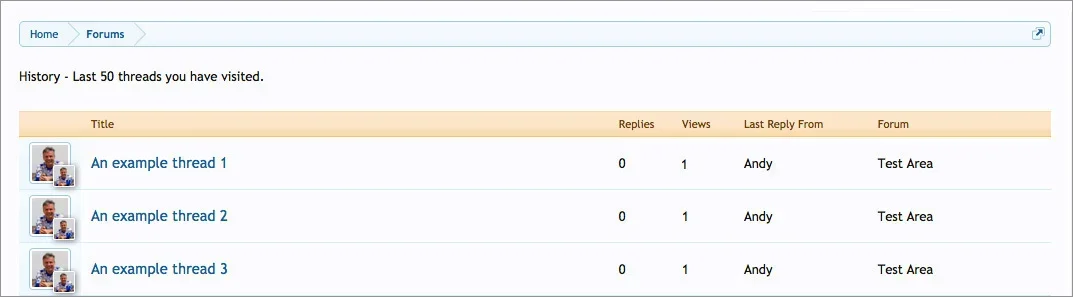