Man, this might be more of a SQL question but I thought I'd give it a shot regardless:
What I want:
Basically, whenever I use the Finder to get a query from the first table (table_note), I want to get the associated values from the second table (note_scribbles) as well.
The first table has an array (note_scribbles) that can store IDs that are related to the IDs of the second table.
Here's the code for both entities:
First table:
Second table:
What my current implementation does:
So whenever I do a query for the first table, it knows that a relation to the second table is there. The array with the IDs is there. But: I receive 'null' as a result when I look at the relation.

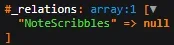
Therefore, I cannot access the second table. I'm not sure what the issue is; my gut feeling says that my structure relations are completely wrong..
Technically, I could use the IDs from the array of the first table and just do another query to get the data from the second table..but that's two queries and I think it's possible to pull all that data at once.
I hope I could explain my problem properly. I'm thankful for any suggestions!
What I want:
Basically, whenever I use the Finder to get a query from the first table (table_note), I want to get the associated values from the second table (note_scribbles) as well.
The first table has an array (note_scribbles) that can store IDs that are related to the IDs of the second table.
Here's the code for both entities:
First table:
PHP:
public static function getStructure(Structure $structure): Structure
{
$structure->table = 'table_note';
$structure->shortName = 'Test\Pad:Note';
$structure->contentType = 'table_note';
$structure->primaryKey = 'id';
$structure->columns = [
'id' => ['type' => self::UINT, 'default' => 0],
'note_scribbles' => ['type' => self::JSON_ARRAY, 'default' => []],
];
$structure->relations = [
'NoteScribbles' => [
'entity' => 'Test\Pad:NoteScribbles',
'type' => self::TO_ONE,
'conditions' => [
[ 'id', '=', ['note_scribbles'] ]
],
// 'primary' => true,
'key' => 'id'
],
];
$structure->defaultWith = [];
$structure->getters = [];
$structure->behaviors = [];
return $structure;
}
Second table:
PHP:
public static function getStructure(Structure $structure): Structure
{
$structure->table = 'table_note_scribbles';
$structure->shortName = 'Test\Pad:NoteScribbles';
$structure->contentType = 'table_note_scribbles';
$structure->primaryKey = 'id';
$structure->columns = [
'id' => ['type' => self::UINT, 'default' => 0],
'content' => ['type' => self::UINT, 'default' => 0],
];
$structure->relations = [
'Note' => [
'entity' => 'Test\Pad:Note',
'type' => self::TO_ONE,
'conditions' => [
[ ['note_scribbles'], '=', 'id' ]
],
// 'primary' => true,
'key' => 'id'
],
];
$structure->defaultWith = [];
$structure->getters = [];
$structure->behaviors = [];
return $structure;
}
What my current implementation does:
So whenever I do a query for the first table, it knows that a relation to the second table is there. The array with the IDs is there. But: I receive 'null' as a result when I look at the relation.

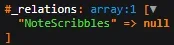
Therefore, I cannot access the second table. I'm not sure what the issue is; my gut feeling says that my structure relations are completely wrong..
Technically, I could use the IDs from the array of the first table and just do another query to get the data from the second table..but that's two queries and I think it's possible to pull all that data at once.
I hope I could explain my problem properly. I'm thankful for any suggestions!