AndyB
Well-known member
Description:
The Similar Threads add-on will show a list of similar threads when a user is creating a new thread.
Key Features:
When a user types a new thread title, as soon as a non-common word is entered a list of similar threads is displayed.
Example:
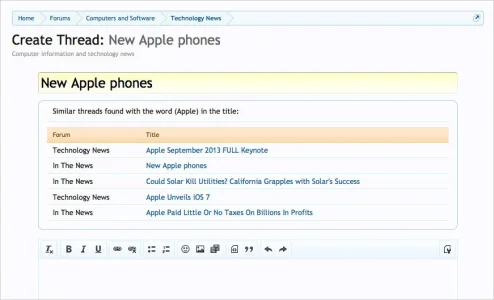
The Similar Threads add-on will show a list of similar threads when a user is creating a new thread.
Key Features:
- Limit number of results (Admin Control Panel Setting)
- Exclude common words (Admin Control Panel Setting)
- Simple one word search
- Node permissions honored
- Similar Threads immediately displayed
- Automatically disabled for displays 800px or less (Responsive design)
- Fully phrased
When a user types a new thread title, as soon as a non-common word is entered a list of similar threads is displayed.
Example:
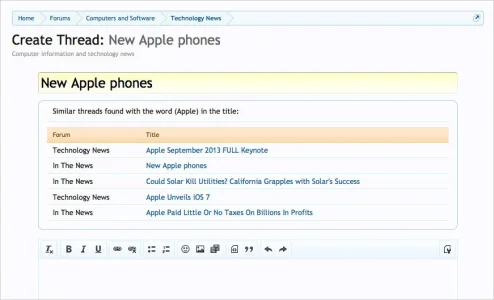