mjda
Well-known member
So I'm wanting to add buttons that will allow members to add larger amounts to the XF number box on a form. I've got the buttons added to the page, but I'm wondering if someone can help me with the JS required to make them work? I have no idea where to even start.
Here's what the form looks like. When they click on +10 or +20 I want it to increase the number in the box by that amount.
Any help on this would be much appreciated.
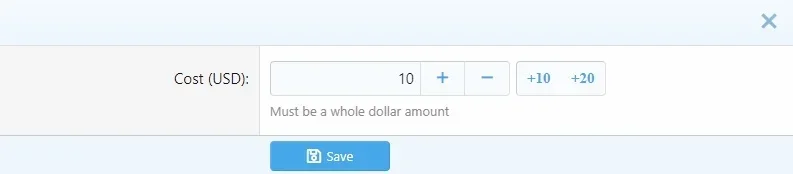
Here's what the form looks like. When they click on +10 or +20 I want it to increase the number in the box by that amount.
Any help on this would be much appreciated.
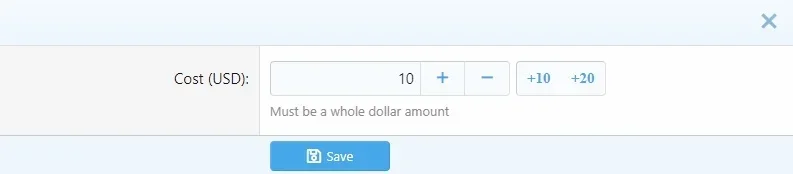