At some point you may want to call an external php file, and have the results show up in your forums. There are a bunch of different ways to do this, but I am going to show a simple example that helps you get the ball rolling.
The example is going to query the XenForo database for all users, and group them by their user group.
Here's what how I would do it.
Create your own directories in your XF library directory.
1. The directory structure should be like this
4. Create a new page node
5. Modify the templates or create a copy
What we are doing here is the public method is called when the page node is created. It will run the two methods
a. getMugList
b. formatMugList
Then we will assign the template name so we can use the pagenode container templatae, more about this later. Finally we will return the html formatted string to be included with the template.
a. getMugList
--------------------------------------
First we are creating an object that will be used to run the query and hold the results.
Next we call the method getMemberList which will run the query
Finally we destroy the object because we no longer need it.
Running the query
--------------------------------------
We created a class in the file QueryUserGroups
The class has one method getMemberList which simply puts the SQL into a string and uses the default XenForo database connection to get the query results.
While it's fetching the results we store them in an array. We are grouping by the user group name for each member. After processing the query we will return the results to the method getMugList, which will store them in static variable.
b. formatMugList
--------------------------------------
Now we will call the method formatMugList. The idea here is to work through the query results and format them into the appropriate html.
First thing we do is set the static variable mugHtml to be blank, this way we can append to the variable and not have any issues.
Next we want to make sure there is something in the array that was returned from the query. If not we will put a default message. This should never happen since you should always have at least 1 member, but in case for some reason the query has an issue.
Next we are going to loop through the array and store the data with html elements into a string. The html is super basic and really doesn't do much. Just for some example purposes.
After we are done looping through the array we exit the method and we are done with the code.
--------------------------------------
Creating the page node
--------------------------------------
Go into the admin control panel.
Click on Display Node Tree icon
And in the top right of the screen click on the create new node button.
Fill out the basic information any way you want.
Here's a screen shot on how I did it.
Click on the page options tab and select if you want to show sibling nodes, child nodes, etc...
Leave the Template HTML box blank
Next click on the PHP Callback tab.
Enter in the name of the class and method.
In our example the class = Example_MembersByUserGroups_MugCallback
and the method = respond
--------------------------------------
Editing the template to display our results
--------------------------------------
There are a few options here.
Here's my preference
XenForo Version 1.2
--------------------------------------
In the admin control panel click on
Appearance
Template modifications
In the top right corner click the create template modification button.
for Template enter in pagenode_container
Search type Simple Replacement
In the Find box enter in <article>{xen:raw $templateHtml}</article>
In the Replace box enter in
$0
<article>{xen:raw $mugHtml}</article>
Save the template modifications.
Now you are done. Test and Enjoy
XenForo Version 1.1.5
--------------------------------------
You have two options either directly edit the template pagenode_container or
you can make a copy of the template and edit the copy.
I would suggest editing a copy of the template, just in case.
When editing the copy find the line that says
<article>{xen:raw $templateHtml}</article>
and replace it with
<article>{xen:raw $mugHtml}</article>
And then in the code find the line that says.
and change it to say
And you should be all set.
--------------------------------------
Notes about this example
--------------------------------------
1. Don't use this example in production if you have a LARGE amount of members on your forum.
2. The query only looks at the primary member group, it needs to be adjusted to look at secondary member groups.
3. Everything listed above is for illustrative purposes only and no warranty is given or implied.
Any questions let me know.
Thanks
--ET
The example is going to query the XenForo database for all users, and group them by their user group.
Here's what how I would do it.
Create your own directories in your XF library directory.
1. The directory structure should be like this
a. /forums/library/Example
b. /forums/library/Example/MembersByUserGroups
2. Write the php filesb. /forums/library/Example/MembersByUserGroups
a. First file is the call back when the page is called, let's call it MugCallback.php
b. Second file is to hold the query, let's call it QueryUserGroups.php
3. Explanation of the Code for the example is belowb. Second file is to hold the query, let's call it QueryUserGroups.php
4. Create a new page node
5. Modify the templates or create a copy
PHP:
public static function respond(XenForo_ControllerPublic_Abstract $controller, XenForo_ControllerResponse_Abstract &$response)
{
self::getMugList();
self::formatMugList();
$response->templateName = "pagenode_container"; //name of the template to use
$response->params['mugHtml'] = self::$mugHtml; //html text to insert into the template
}
What we are doing here is the public method is called when the page node is created. It will run the two methods
a. getMugList
b. formatMugList
Then we will assign the template name so we can use the pagenode container templatae, more about this later. Finally we will return the html formatted string to be included with the template.
a. getMugList
--------------------------------------
PHP:
private static function getMugList()
{
$userList = new Example_MembersByUserGroups_QueryUserGroups();
self::$mugList = $userList->getMemberList();
unset($userList);
}
Next we call the method getMemberList which will run the query
Finally we destroy the object because we no longer need it.
Running the query
--------------------------------------
We created a class in the file QueryUserGroups
PHP:
class Example_MembersByUserGroups_QueryUserGroups extends XenForo_Model
{
public function getMemberList()
{
//exclude guest group, default group id for guest group is 0
//exclude members who have been banned
$sql_query = "SELECT g.title AS 'user_group_name'
,u.user_id
,u.username
FROM xf_user_group AS g
INNER JOIN xf_user AS u
ON g.user_group_id = u.user_group_id
WHERE g.user_group_id > 0
AND u.is_banned = 0 ";
$memberlist = $this->_getDb()->query($sql_query);
while ($qry = $memberlist->fetch())
{
$qryresult[$qry['user_group_name']][$qry['user_id']] = $qry['username'];
}
return ($qryresult);
}
}
The class has one method getMemberList which simply puts the SQL into a string and uses the default XenForo database connection to get the query results.
While it's fetching the results we store them in an array. We are grouping by the user group name for each member. After processing the query we will return the results to the method getMugList, which will store them in static variable.
b. formatMugList
--------------------------------------
Now we will call the method formatMugList. The idea here is to work through the query results and format them into the appropriate html.
PHP:
private static function formatMugList()
{
self::$mugHtml = ""; //set the variable to a blank value
//make sure the query returned an array and there is 1 value in the array
if(is_array(self::$mugList) && count(self::$mugList) > 0)
{
//loop through each member group
foreach (self::$mugList as $groupName=>$memberList)
{
self::$mugHtml .= $groupName . " <br />";
//loop through each member name
foreach ($memberList as $member)
{
self::$mugHtml .= "<li>" . $member . "</li>";
}
}
}
else
{
// if some reason the query didn't work output a message to user
self::$mugHtml = "<div> No members found </div>";
return;
}
self::$mugHtml = '<ul>' . self::$mugHtml . '</ul>';
}
Next we want to make sure there is something in the array that was returned from the query. If not we will put a default message. This should never happen since you should always have at least 1 member, but in case for some reason the query has an issue.
Next we are going to loop through the array and store the data with html elements into a string. The html is super basic and really doesn't do much. Just for some example purposes.
After we are done looping through the array we exit the method and we are done with the code.
--------------------------------------
Creating the page node
--------------------------------------
Go into the admin control panel.
Click on Display Node Tree icon
And in the top right of the screen click on the create new node button.
Fill out the basic information any way you want.
Here's a screen shot on how I did it.
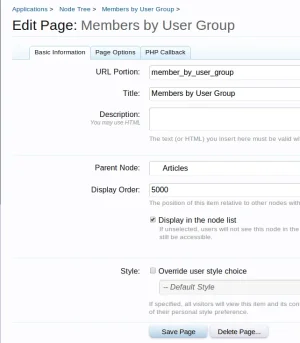
Click on the page options tab and select if you want to show sibling nodes, child nodes, etc...
Leave the Template HTML box blank
Next click on the PHP Callback tab.
Enter in the name of the class and method.
In our example the class = Example_MembersByUserGroups_MugCallback
and the method = respond
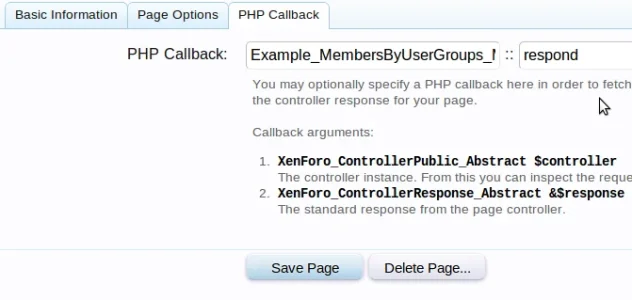
--------------------------------------
Editing the template to display our results
--------------------------------------
There are a few options here.
Here's my preference
XenForo Version 1.2
--------------------------------------
In the admin control panel click on
Appearance
Template modifications
In the top right corner click the create template modification button.
for Template enter in pagenode_container
Search type Simple Replacement
In the Find box enter in <article>{xen:raw $templateHtml}</article>
In the Replace box enter in
$0
<article>{xen:raw $mugHtml}</article>
Save the template modifications.
Now you are done. Test and Enjoy
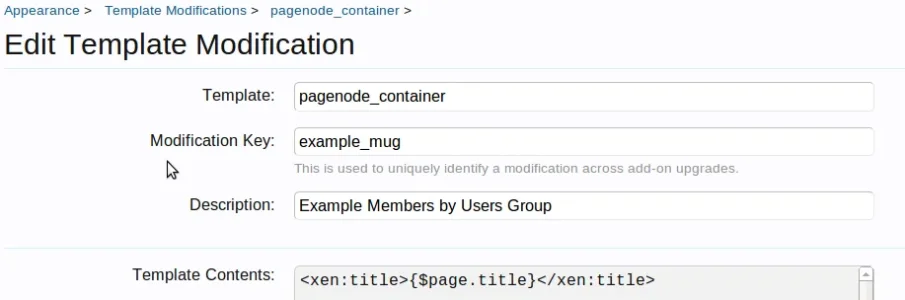
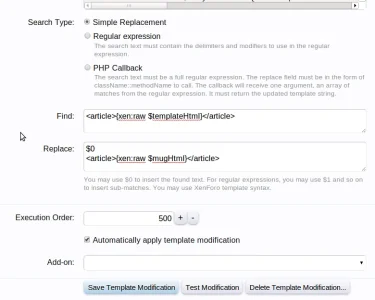
XenForo Version 1.1.5
--------------------------------------
You have two options either directly edit the template pagenode_container or
you can make a copy of the template and edit the copy.
I would suggest editing a copy of the template, just in case.
When editing the copy find the line that says
<article>{xen:raw $templateHtml}</article>
and replace it with
<article>{xen:raw $mugHtml}</article>
And then in the code find the line that says.
PHP:
$response->templateName = "pagenode_container"; //name of the template to use
PHP:
$response->templateName = "copy_of_pagenode_container"; //name of the template to use
And you should be all set.
--------------------------------------
Notes about this example
--------------------------------------
1. Don't use this example in production if you have a LARGE amount of members on your forum.
2. The query only looks at the primary member group, it needs to be adjusted to look at secondary member groups.
3. Everything listed above is for illustrative purposes only and no warranty is given or implied.
Any questions let me know.
Thanks
--ET